vue Syntax Error: Error: PostCSS plugin postcss-pxtorem requires PostCSS 8.
migration guide for end users:
for the scaffold generation project, configure the adaptive postcss pxtorem configuration and report an error
First of all, you are postcssrc. JS file normal configuration (detailed configuration)
module.exports = {
plugins: {
autoprefixer: {
overrideBrowserslist: [
"Android 4.1",
"iOS 7.1",
"Chrome > 31",
"ff > 31",
"ie >= 8",
"last 10 versions",
],
grid: true,
},
"postcss-pxtorem": {
rootValue: 37.5, //The result is: design element size / 16, for example, element width 320px, the final page will be converted to 20rem
propList: ["*"], // is a list of properties that will be converted, here set to ['*'] all, suppose you need to set only the border, you can write ['*', '!border*']
unitPrecision: 5, //how many decimal places to keep rem
//selectorBlackList: ['.radius'], //then it is an array for filtering css selectors, for example you set it to ['fs'], that for example fs-xl class name, inside the style about px will not be converted, here also support regular writing.
replace: true, //I really don't know what this is for. If you know, let me know!
mediaQuery: false, //media query (@media screen or something like that) does not work
minPixelValue: 12, //px less than 12 will not be converted
},
},
};
You can also do this (a little simpler)
module.exports = {
plugins: {
'autoprefixer': {
browsers: ['Android >= 4.0', 'iOS >= 8']
},
'postcss-pxtorem': {
rootValue: 37.5,
propList: ['*']
}
}
}
At this time, if the project is configured normally, there will be an error in running the project
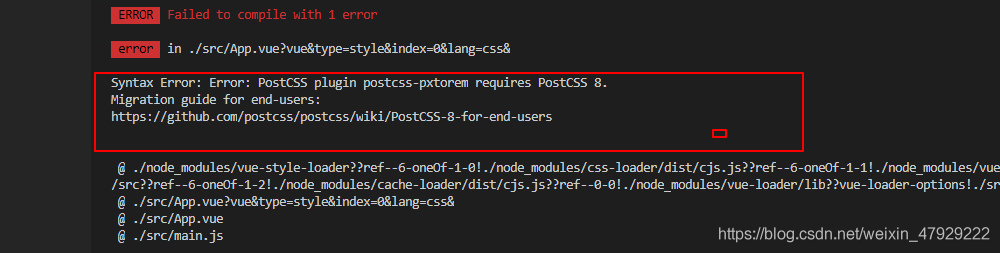
It is said that your postcss can not identify the postcss-pxtorem , so the error is caused, this time you can not configure the postcssrc.js, only the comment configuration will work properly, this time you can package.json to look at your dependencies
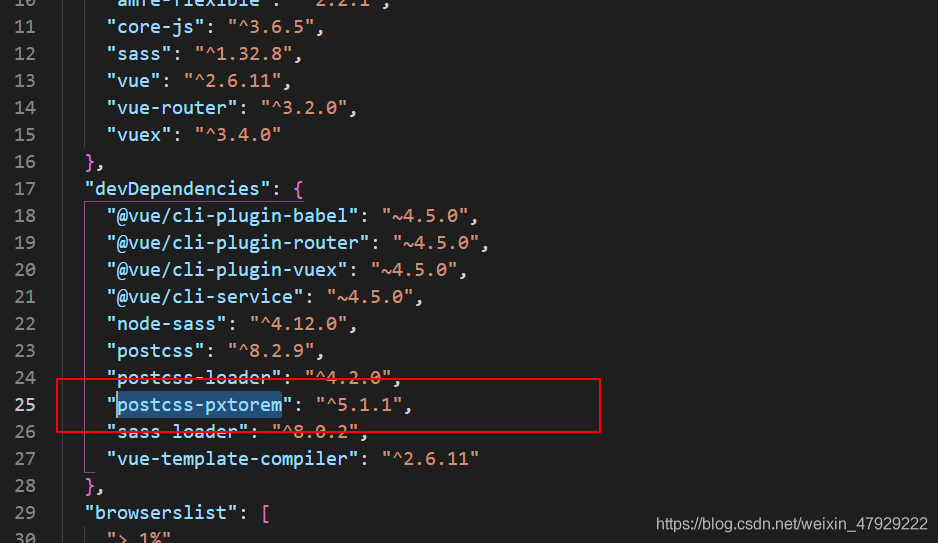
At this time you will find that your postcss-pxtorem is 6.xxx version, because it is his version is too high, so the problem is caused
You just need to lower the version to 5.1.1 on OK
npm i [email protected]
Then you run it again to solve the problem