Problem description
When using the python SDK, when logging in to China azure (mooncake) and accessing the alertsmanagement resources, you often encounter the error message “environmentcredential: authentication failed”.
Python code:
from azure.identity import DefaultAzureCredential
from azure.mgmt.alertsmanagement import AlertsManagementClient
# Acquire a credential object using CLI-based authentication.
credential = DefaultAzureCredential()
subscription_id = "xxxx-xxxx-xxxx-xxxx-xxxx"
alertClient = AlertsManagementClient(credential,subscription_id,base_url="https://management.chinacloudapi.cn/")
rules = alertClient.smart_detector_alert_rules.list()
for rule in rules:
print("Rule Name: " + rule.name)
Error message:
PS C:\LBWorkSpace\MyCode\46-alertrule-python> python getrule.py
DefaultAzureCredential failed to retrieve a token from the included credentials.
Attempted credentials:
EnvironmentCredential: Authentication failed: AADSTS500011: The resource principal named https://management.azure.com was not found in the tenant named xxx Mooncake. This can happen if the application has not been installed by the administrator of the tenant or consented to by any user in the tenant. You might have sent your authentication request to the wrong tenant.
Trace ID: xxxxxxxx-xxxx-xxxx-xxxx-9e130dbf7900
Correlation ID: xxxxxxxx-xxxx-xxxx-xxxx-46769c9e1e10
Timestamp: 2022-01-27 12:09:35Z
To mitigate this issue, please refer to the troubleshooting guidelines here at https://aka.ms/azsdk/python/identity/defaultazurecredential/troubleshoot.
Traceback (most recent call last):
File "C:\LBWorkSpace\MyCode\46-alertrule-python\getrule.py", line 15, in <module>
for rule in rules:
File "C:\Users\bulu\AppData\Local\Programs\Python\Python310\lib\site-packages\azure\core\paging.py", line 129, in __next__
return next(self._page_iterator)
File "C:\Users\bulu\AppData\Local\Programs\Python\Python310\lib\site-packages\azure\core\paging.py", line 76, in __next__
self._response = self._get_next(self.continuation_token)
File "C:\Users\bulu\AppData\Local\Programs\Python\Python310\lib\site-packages\azure\core\pipeline\policies\_redirect.py", line 158, in send
response = self.next.send(request)
File "C:\Users\bulu\AppData\Local\Programs\Python\Python310\lib\site-packages\azure\core\pipeline\policies\_retry.py", line 445, in send
response = self.next.send(request)
File "C:\Users\bulu\AppData\Local\Programs\Python\Python310\lib\site-packages\azure\core\pipeline\policies\_authentication.py", line 117, in send
self.on_request(request)
File "C:\Users\bulu\AppData\Local\Programs\Python\Python310\lib\site-packages\azure\core\pipeline\policies\_authentication.py", line 94, in on_request
self._token = self._credential.get_token(*self._scopes)
File "C:\Users\bulu\AppData\Local\Programs\Python\Python310\lib\site-packages\azure\identity\_credentials\default.py", line 172, in get_token
return super(DefaultAzureCredential, self).get_token(*scopes, **kwargs)
File "C:\Users\bulu\AppData\Local\Programs\Python\Python310\lib\site-packages\azure\identity\_credentials\chained.py", line 108, in get_token
raise ClientAuthenticationError(message=message)
azure.core.exceptions.ClientAuthenticationError: DefaultAzureCredential failed to retrieve a token from the included credentials.
Attempted credentials:
EnvironmentCredential: Authentication failed: AADSTS500011: The resource principal named https://management.azure.com was not found in the tenant named xxxx Mooncake. This can happen if the application has not been installed by the administrator of the tenant or consented to by any user in the tenant. You might have sent your authentication request to the wrong tenant.
Trace ID: xxxxxxxx-xxxx-xxxx-xxxx-9e130dbf7900
Correlation ID: xxxxxxxx-xxxx-xxxx-xxxx-46769c9e1e10
Timestamp: 2022-01-27 12:09:35Z
To mitigate this issue, please refer to the troubleshooting guidelines here at https://aka.ms/azsdk/python/identity/defaultazurecredential/troubleshoot.
Problem-solving:
From the error message https://management.azure.com, we know that the problem is due to the default value of Resource Principal used in AlertsManagementClient, which was not changed to base_url with the specification of
https://management.chinacloudapi.cn/ . The problem can be mitigated by specifying credential_scopes as [“https://management.chinacloudapi.cn/.default”] when constructing the AlertsManagementClient object.
The modified code is :
# pre:
alertClient = AlertsManagementClient(credential,subscription_id,base_url="https://management.chinacloudapi.cn/")
# new:
alertClient = AlertsManagementClient(credential,subscription_id,base_url="https://management.chinacloudapi.cn/",credential_scopes=["https://management.chinacloudapi.cn/.default"])
PS: when creating client objects of other resources, if you encounter the same principal problem, you can set credential_Scopes to solve the problem.
The complete code that can travel far is:
# Import the needed credential and management objects from the libraries.
from azure.identity import DefaultAzureCredential
from azure.mgmt.alertsmanagement import AlertsManagementClient
# Acquire a credential object using CLI-based authentication.
credential = DefaultAzureCredential()
subscription_id = "a9dc7515-7692-4316-9ad4-762f383eec10"
# # pre:
# alertClient = AlertsManagementClient(credential,subscription_id,base_url="https://management.chinacloudapi.cn/")
# Modified:
alertClient = AlertsManagementClient(credential,subscription_id,base_url="https://management.chinacloudapi.cn/",credential_scopes=["https://management.chinacloudapi.cn/.default"])
rules = alertClient.smart_detector_alert_rules.list()
for rule in rules:
print("Rule Name: " + rule.name)
Operation results:
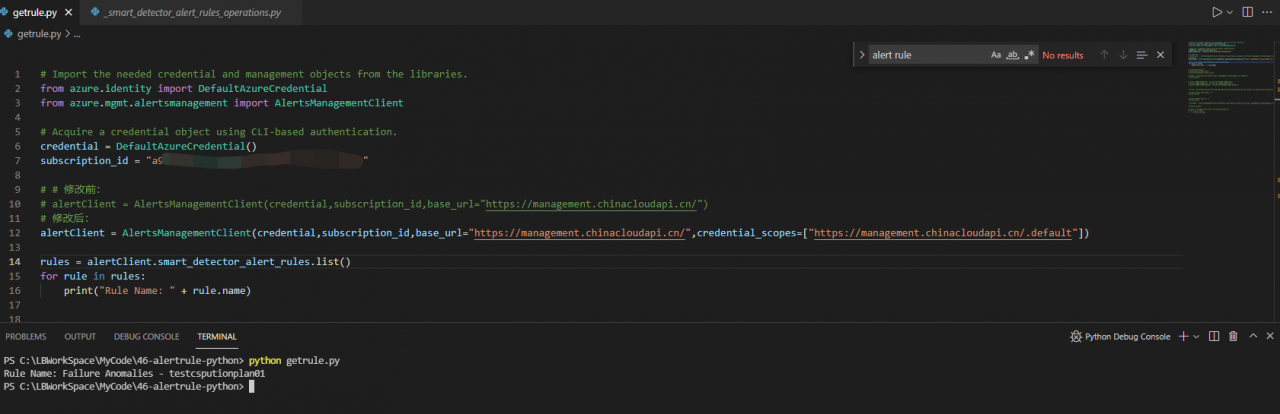
The correct MonitorManagementClient object to get metric_alerts and activity_log_alerts Get Alert Rule code
from azure.mgmt.monitor import MonitorManagementClient
from azure.identity import DefaultAzureCredential
from msrestazure.azure_cloud import AZURE_CHINA_CLOUD as CLOUD
import os
os.environ["SUBSCRIPTION_ID"] = "xxxxxxyour-subidxxxxxx"
os.environ["AZURE_TENANT_ID"] = "your tenant idxxxxx"
os.environ["AZURE_CLIENT_ID"] = "client_id_sp"
os.environ["AZURE_CLIENT_SECRET"] = "pw_sp"
subscription_id = os.environ["SUBSCRIPTION_ID"]
credential = DefaultAzureCredential(authority=CLOUD.endpoints.active_directory)
# create client
client1 = MonitorManagementClient(
credential,
subscription_id,
base_url=CLOUD.endpoints.resource_manager,
credential_scopes=[CLOUD.endpoints.resource_manager + "/.default"]
)
#classic
my_alerts1 = client1.alert_rules.list_by_subscription()
for j in my_alerts1:
print(j)
#log search alerts
client2 = MonitorManagementClient(
credential,
subscription_id,
base_url=CLOUD.endpoints.resource_manager,
credential_scopes=[CLOUD.endpoints.resource_manager + "/.default"]
)
my_alerts2 = client2.scheduled_query_rules.list_by_subscription()
for j in my_alerts2:
print(j)
#activity alerts
client3 = MonitorManagementClient(
credential,
subscription_id,
base_url=CLOUD.endpoints.resource_manager,
credential_scopes=[CLOUD.endpoints.resource_manager + "/.default"],
api_version="2017-04-01"
)
my_alerts3 = client3.activity_log_alerts.list_by_subscription_id()
for j in my_alerts3:
print(j)
#metric alerts
client4 = MonitorManagementClient(
credential,
subscription_id,
base_url=CLOUD.endpoints.resource_manager,
credential_scopes=[CLOUD.endpoints.resource_manager + "/.default"]
)
my_alerts4 = client4.metric_alerts.list_by_subscription()
for j in my_alerts4:
print(j)
Outcome:
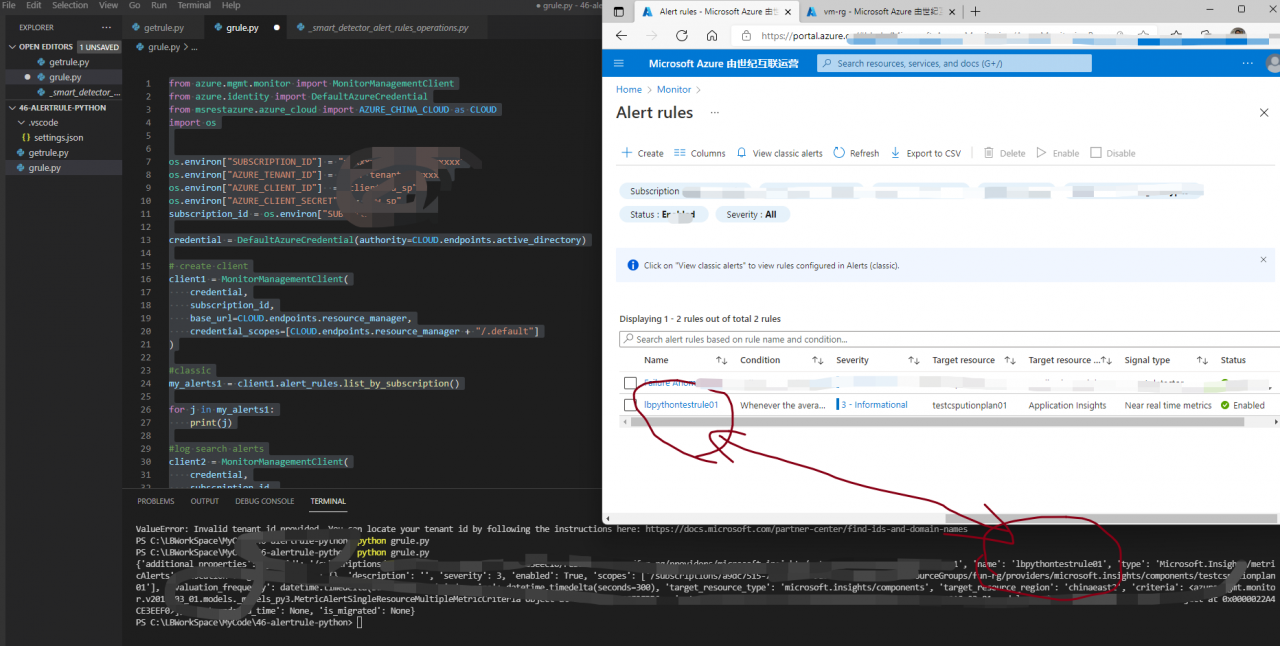