PDO statement:: bindparam vs bindvalue technology of PHP extending PDO MySQL
May yes published on November 16, 2015 13:27
Link to the original text: http://blog.lmlphp.com/archives/155/The_ difference_ of_ PDOStatement_ bindParam_ and_ bindValue_ of_ PHP_ extension_ PDO_ MySQL from lmlphp backyard
Lblog online experience site will be launched a few days ago http://lblog.lmlphp.com/ It’s been months since I moved to VPS. The version of PHP on the new VPS is relatively high, so when running, there will be an obsolete error of MySQL functions. The easiest way to deal with this error is to block it at the error reporting level, but this is not my style. I hope to solve it in a better way. But when I go to work, I don’t have time to write a class of mysqlpdo enhance, according to the style of MySQL driver class in lmlphp. For this reason, when writing PDO operation class, I specially defined an interface to restrict my behavior for fear of problems. After testing, it was proved that it was completely compatible with the previous MySQL class. It took so many months to finish writing, and I really couldn’t afford to hurt
This time when writing PDO driver class, I didn’t refer to other people’s writing method. I completely looked at the official documents, combined with my own needs, and tried to realize it as simple as possible. In fact, PDO is already an object-oriented style. In fact, it doesn’t need any driver class to encapsulate too much. It’s just written for better compatibility with the code in the project. At the beginning, when I looked at bindvalue and bindparam, the document gave me the feeling that one was a variable and the other was the exact value. It was only later found that one was a reference and the other was a common parameter. When testing the modification operation, it was found that the last field in the database was the same as the previous string, and the int type was not affected. Maybe my brain was too tired at that time. I worked out this problem for a long time. The next day I found out that it was caused by using bindparam in the loop
The mysqlpdoenhance driver class has been uploaded to lmlphp and lblog. Lblog has automatically selected the corresponding driver class when initializing the instance. This improvement makes the lblog system more excellent and more adaptable to the server environment
Mysqlpdoenhance class continues the simple style of MySQL class, with only one heavyweight query method to automatically determine whether to return resources or the number of rows affected. At the same time, we find that we can’t do this well when we use PDO. Because the number of rows is also affected when select is executed, which is different from common sense. People who understand databases should know that select does not affect rows, but the value returned by the rowcount method in PDO is the number of rows selected. Therefore, for this reason, we can only make a simple judgment from SQL, which is not perfect
An extract of query method is attached
public function query($sql, $params = array()){ $stmt = $this->db->prepare($sql, array(PDO::MYSQL_ATTR_USE_BUFFERED_QUERY => true)); if($params){ foreach ($params as $k => $v){ if(is_array($v)){ $value = $v['value']; $type = isset($v['type']) ?$v['type'] : false; $length = isset($v['length']) ?$v['length'] : false; if($type && $length){ $stmt->bindValue($k, $value, $type, $length); }elseif($type){ $stmt->bindValue($k, $value, $type); }else{ $stmt->bindValue($k, $value); } }else{ $stmt->bindValue($k, $v); } } } $stmt->execute(); if(preg_match('/^update|^insert/i', trim($sql))){ return $stmt->rowCount(); }else{ return $stmt->fetchAll(PDO::FETCH_ASSOC); }}
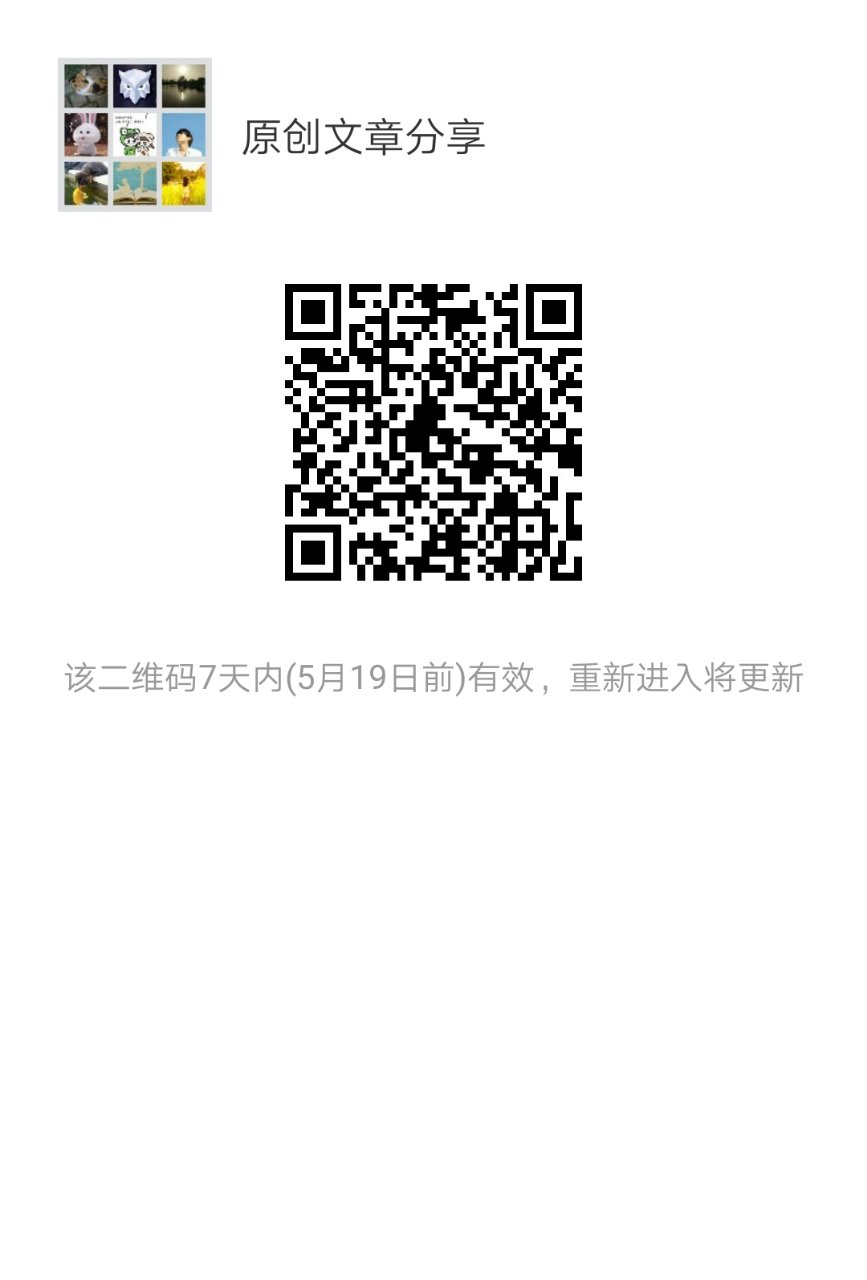
Read (606) comments (0) view comments