Ie page blank
Error message


The page is blank
Error reporting reason
Babel only converts new JavaScript syntax (such as arrow functions) by default, rather than new APIs, such as iterator, generator, set, maps, proxy, reflect, symbol, promise and other new objects or methods
For example, promise, new native methods such as string. Padstart (left pad), etc. To solve this problem, we use a technology called Polyfill
In short, the compatibility problem is that ie does not support the syntax of some new objects and expressions in ES6. The solution is to use Babel Polyfill to use ES6 normally
What is Polyfill
For example, some old browsers do not support the number.isnan method. Polyfill can be as follows:
if(!Number.isNaN) {
Number.isNaN = function(num) {
return(num !== num);
}
}
Polyfill solves the API compatibility problem in a similar way
Solution
1. Using Babel Polyfill
Introducing Babel Polyfill
npm i -S babel-polyfill
How to use
It needs to be introduced at the top of your application’s entry point or in the packaging configuration
Introduce in main.js
import 'babel-polyfill'
Or modify the configuration in webpack.base.conf.js in config
entry:{
app:['babel-polyfill','./src/main.js']
}
2. Add the following code to index.html (not required)
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
3. Module transcoding specified in Babel loader
Whether poly fill is added or an error is reported. Generally, third-party UI frameworks, libraries and plug-ins are used, and the underlying syntax of these UI frameworks, libraries and plug-ins is ES6
Locate the Babel loader in webpack.base.conf.js
For example, I use element UI and v-charts here Amend to read as follows:
{
test: /\.js$/,
loader: 'babel-loader',
include: [
resolve('src'),
resolve('test'),
resolve('node_modules/webpack-dev-server/client'),
resolve('node_modules/v-charts/src'),
resolve('node_modules/vue-awesome'),
resolve('node_modules/element-ui/packages'),
resolve('node_modules/element-ui/src')
]
}
4. Packaging problem
If there is a webpack code packaging error, generally go to GitHub webpack issues
This error is due to the webpack dev server version problem
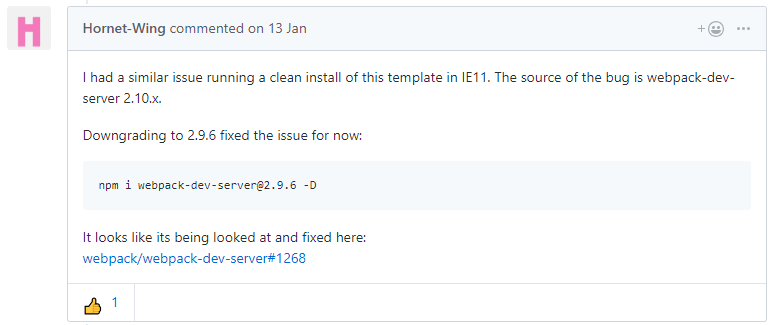
5. Babel configuration. Babelrc file
. babelrc is the configuration file of Babel, which is placed in the root directory of the project. Generally, the. Babelrc file does not need to be modified. If you do all the above and still report an error, you can view the official website document to configure the file
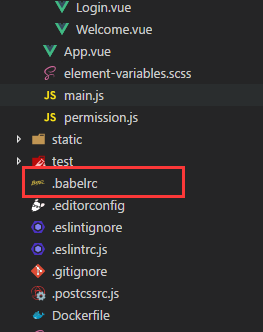
In the code generated by the project using Vue cli, there is a. Babelrc file in the root directory. In the default generated template content, add “usebuiltins”: “entry” , This is a setting that specifies which content needs to be Polyfill (compatible).
usebuiltins there are three settings
False – do nothing
Entry – according to the support of the browser version, the Polyfill requirements are split and imported, and only Polyfill that is not supported by the browser is imported
Usage – check the usage of ES6/7/8 in the code, and only load the Polyfill used in the code
{
"presets": [
["env", {
"modules": false,
"useBuiltIns": "entry"
}],
"stage-2"
],
"plugins": ["transform-runtime", "transform-vue-jsx"],
"env": {
"test": {
"presets": ["env", "stage-2"],
"plugins": ["istanbul"]
}
}
}
See Babel’s official website for details: https://www.babeljs.cn/
Summary
1. Projects written in Vue can be compatible with IE9 and above at most
2. Use babel-polyfill
3. Specify the UI framework, library and plug-in path of the third party to be transcoded in Babel loader
4. Configure. Babelrc file
5. Babel is a powerful thing https://www.babeljs.cn/