When using pipenv shell to switch to virtual environment, an error is reported: attributeerror: ‘module’ object has no attribute ‘run’
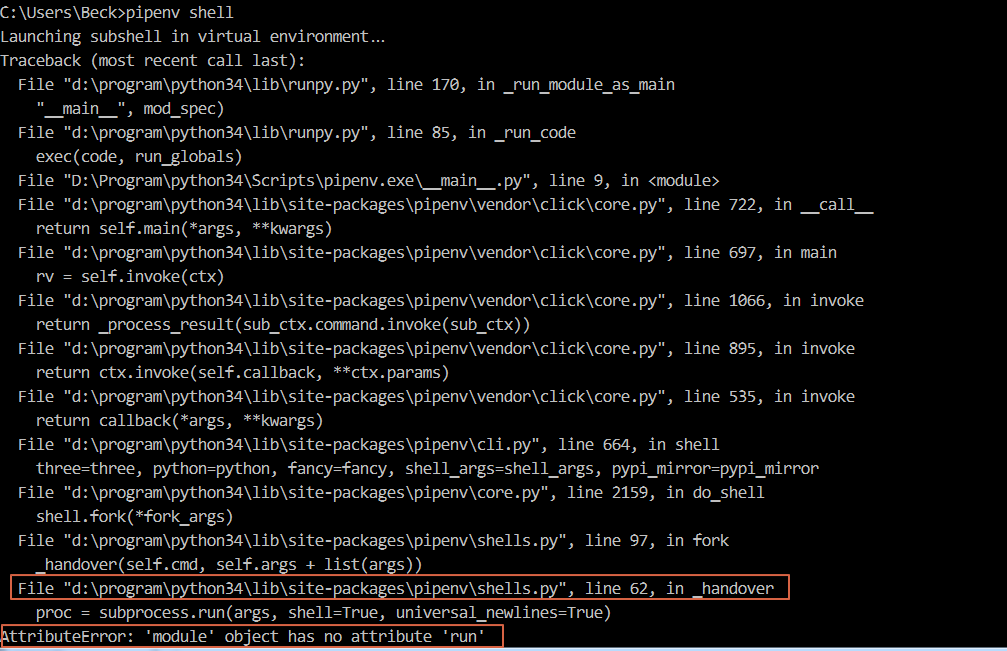
You can see that there is an error in line 62 of the file D:: (program) python34 (LIB) site packages (pipenv) shells. Py, which indicates that the module does not have the property of run, so you go to line 62 of the file to see it
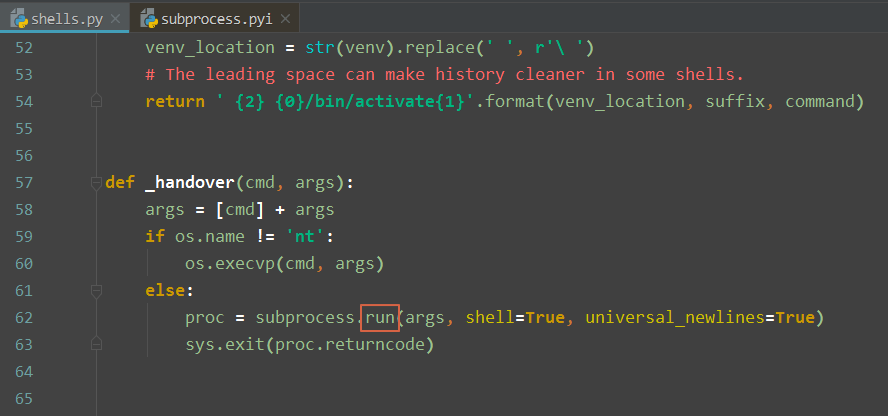
Select run and Ctrl + B to find the source code. The source code is as follows:
if sys.version_info >= (3, 6):
# Nearly same args as Popen.__init__ except for timeout, input, and check
def run(args: _CMD,
timeout: Optional[float] = ...,
input: Optional[_TXT] = ...,
check: bool = ...,
bufsize: int = ...,
executable: _PATH = ...,
stdin: _FILE = ...,
stdout: _FILE = ...,
stderr: _FILE = ...,
preexec_fn: Callable[[], Any] = ...,
close_fds: bool = ...,
shell: bool = ...,
cwd: Optional[_PATH] = ...,
env: Optional[_ENV] = ...,
universal_newlines: bool = ...,
startupinfo: Any = ...,
creationflags: int = ...,
restore_signals: bool = ...,
start_new_session: bool = ...,
pass_fds: Any = ...,
*,
encoding: Optional[str] = ...,
errors: Optional[str] = ...) -> CompletedProcess: ...
else:
# Nearly same args as Popen.__init__ except for timeout, input, and check
def run(args: _CMD,
timeout: Optional[float] = ...,
input: Optional[_TXT] = ...,
check: bool = ...,
bufsize: int = ...,
executable: _PATH = ...,
stdin: _FILE = ...,
stdout: _FILE = ...,
stderr: _FILE = ...,
preexec_fn: Callable[[], Any] = ...,
close_fds: bool = ...,
shell: bool = ...,
cwd: Optional[_PATH] = ...,
env: Optional[_ENV] = ...,
universal_newlines: bool = ...,
startupinfo: Any = ...,
creationflags: int = ...,
restore_signals: bool = ...,
start_new_session: bool = ...,
pass_fds: Any = ...) -> CompletedProcess: ...
But look at the first line, if sys. Version_ info >= ( 3, 6): let’s guess for a moment. This 3.6 is the version number of Python. If the version is greater than 3.6, use the first run function, otherwise use the second run function. What’s the difference between the two functions?The first run function has two more lines
encoding: Optional[str] = ...,
errors: Optional[str] = ...)
However, since the version greater than 3.6 and less than 3.6 will call the existing run function, why will an error be reported?Let’s take a look at the more complete code
I don’t know if I have noticed that when the version is greater than 3.5, I will go to the second if to judge whether it is greater than 3.6. However, my current version is 3.4.4, so I don’t satisfy the if judgment greater than 3.5, but satisfy the if judgment greater than 3.3. In this case, the following methods are all call (in fact, as long as the version & lt; 3.5, call method should be called)
if sys.version_info >= (3, 5):
class CompletedProcess:
# morally: _CMD
args = ... # type: Any
returncode = ... # type: int
# morally: Optional[_TXT]
stdout = ... # type: Any
stderr = ... # type: Any
def __init__(self, args: _CMD,
returncode: int,
stdout: Optional[_TXT] = ...,
stderr: Optional[_TXT] = ...) -> None: ...
def check_returncode(self) -> None: ...
if sys.version_info >= (3, 6):
# Nearly same args as Popen.__init__ except for timeout, input, and check
def run(args: _CMD,
timeout: Optional[float] = ...,
input: Optional[_TXT] = ...,
check: bool = ...,
bufsize: int = ...,
executable: _PATH = ...,
stdin: _FILE = ...,
stdout: _FILE = ...,
stderr: _FILE = ...,
preexec_fn: Callable[[], Any] = ...,
close_fds: bool = ...,
shell: bool = ...,
cwd: Optional[_PATH] = ...,
env: Optional[_ENV] = ...,
universal_newlines: bool = ...,
startupinfo: Any = ...,
creationflags: int = ...,
restore_signals: bool = ...,
start_new_session: bool = ...,
pass_fds: Any = ...,
*,
encoding: Optional[str] = ...,
errors: Optional[str] = ...) -> CompletedProcess: ...
else:
# Nearly same args as Popen.__init__ except for timeout, input, and check
def run(args: _CMD,
timeout: Optional[float] = ...,
input: Optional[_TXT] = ...,
check: bool = ...,
bufsize: int = ...,
executable: _PATH = ...,
stdin: _FILE = ...,
stdout: _FILE = ...,
stderr: _FILE = ...,
preexec_fn: Callable[[], Any] = ...,
close_fds: bool = ...,
shell: bool = ...,
cwd: Optional[_PATH] = ...,
env: Optional[_ENV] = ...,
universal_newlines: bool = ...,
startupinfo: Any = ...,
creationflags: int = ...,
restore_signals: bool = ...,
start_new_session: bool = ...,
pass_fds: Any = ...) -> CompletedProcess: ...
# Same args as Popen.__init__
if sys.version_info >= (3, 3):
# 3.3 added timeout
def call(args: _CMD,
bufsize: int = ...,
executable: _PATH = ...,
stdin: _FILE = ...,
stdout: _FILE = ...,
stderr: _FILE = ...,
preexec_fn: Callable[[], Any] = ...,
close_fds: bool = ...,
shell: bool = ...,
cwd: Optional[_PATH] = ...,
env: Optional[_ENV] = ...,
universal_newlines: bool = ...,
startupinfo: Any = ...,
creationflags: int = ...,
restore_signals: bool = ...,
start_new_session: bool = ...,
pass_fds: Any = ...,
timeout: float = ...) -> int: ...
else:
def call(args: _CMD,
bufsize: int = ...,
executable: _PATH = ...,
stdin: _FILE = ...,
stdout: _FILE = ...,
stderr: _FILE = ...,
preexec_fn: Callable[[], Any] = ...,
close_fds: bool = ...,
shell: bool = ...,
cwd: Optional[_PATH] = ...,
env: Optional[_ENV] = ...,
universal_newlines: bool = ...,
startupinfo: Any = ...,
creationflags: int = ...,
restore_signals: bool = ...,
start_new_session: bool = ...,
pass_fds: Any = ...) -> int: ...
If you understand this, you will know how to solve it. Just modify the source code and change run to call (if you want to use version 3.5 or above, it is recommended to change call back to run)
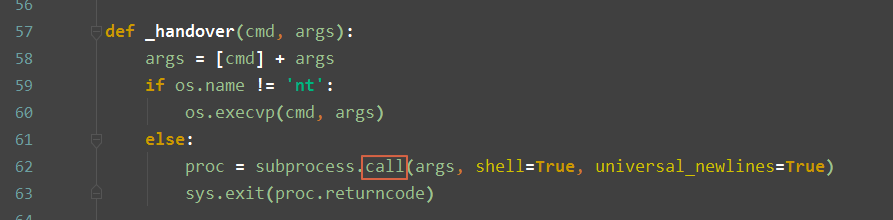
Using the pipenv shell again, you can switch to the virtual environment
