How to solve python Installation WARNING: pip is configured with locations that require TLS/SSL, however the ssl module in Python is not available.
WARNING: pip is configured with locations that require TLS/SSL, however the ssl module in Python is not available.
1. Install the relevant dependencies
yum install gcc libffi-devel zlib* openssl-devel
# libffi-devel zlib-devel bzip2-devel openssl-devel ncurses-devel sqlite-devel readline-devel tk-devel gcc make
2. Download and unzip
wget https://www.python.org/ftp/python/3.7.1/Python-3.7.1.tar.xz
# Download
wget https://www.python.org/ftp/python/3.7.1/Python-3.7.1.tar.xz
# Unzip and compile.
tar -xvJf Python-3.7.1.tar.xz
cd Python-3.7.1
3. Compile and install
. /configure prefix=/usr/local/python3
make && make install
# After compiling, create a softlink file to the executable path.
ln -s /usr/local/python3/bin/python3 /usr/bin/python3
ln -s /usr/local/python3/bin/pip3 /usr/bin/pip3
# We can clear the previously compiled executables and configuration files && Clear all generated files:.
make clean && make distclean
bug: Failed to use pip command
2.1 Error messages
pip is configured with locations that require TLS/SSL, however the ssl module in Python is not available.
Collecting virtualenv
Retrying (Retry(total=4, connect=None, read=None, redirect=None, status=None)) after connection broken by ‘SSLError(“Can’t connect to HTTPS URL because the SSL module is not available.”)’: /simple/virtualenv/
Retrying (Retry(total=3, connect=None, read=None, redirect=None, status=None)) after connection broken by ‘SSLError(“Can’t connect to HTTPS URL because the SSL module is not available.”)’: /simple/virtualenv/
Retrying (Retry(total=2, connect=None, read=None, redirect=None, status=None)) after connection broken by ‘SSLError(“Can’t connect to HTTPS URL because the SSL module is not available.”)’: /simple/virtualenv/
Retrying (Retry(total=1, connect=None, read=None, redirect=None, status=None)) after connection broken by ‘SSLError(“Can’t connect to HTTPS URL because the SSL module is not available.”)’: /simple/virtualenv/
Retrying (Retry(total=0, connect=None, read=None, redirect=None, status=None)) after connection broken by ‘SSLError(“Can’t connect to HTTPS URL because the SSL module is not available.”)’: /simple/virtualenv/
Could not fetch URL https://pypi.org/simple/virtualenv/: There was a problem confirming the ssl certificate: HTTPSConnectionPool(host=’pypi.org’, port=443): Max retries exceeded with url: /simple/virtualenv/ (Caused by SSLError(“Can’t connect to HTTPS URL because the SSL module is not available.”)) – skipping
Could not find a version that satisfies the requirement virtualenv (from versions: )
No matching distribution found for virtualenv
pip is configured with locations that require TLS/SSL, however the ssl module in Python is not available.
Could not fetch URL https://pypi.org/simple/pip/: There was a problem confirming the ssl certificate: HTTPSConnectionPool(host=’pypi.org’, port=443): Max retries exceeded with url: /simple/pip/ (Caused by SSLError(“Can’t connect to HTTPS URL because the SSL module is not available.”)) – skipping
2.2 Reason
System version centos6.5, where openssl version is OpenSSL 1.0.1e-fips 11 Feb 2013, and python3.7 needs openssl version 1.0.2 or 1.1.x, need to upgrade openssl and recompile python3.7.0. The version of openssl installed by yum is relatively low.
2.3 Upgrade openssl
# 1. download openssl
wget https://www.openssl.org/source/openssl-1.1.1a.tar.gz
tar -zxvf openssl-1.1.1a.tar.gz
cd openssl-1.1.1a
# 2.Compile and install
. /config –prefix=/usr/local/openssl no-zlib #no-zlib
make
make install
# 3.Back up the original configuration
mv /usr/bin/openssl /usr/bin/openssl.bak
mv /usr/include/openssl/ /usr/include/openssl.bak
# 4. New version configuration
ln -s /usr/local/openssl/include/openssl /usr/include/openssl
ln -s /usr/local/openssl/lib/libssl.so.1.1 /usr/local/lib64/libssl.so
ln -s /usr/local/openssl/bin/openssl /usr/bin/openssl
# 5. Modify the system configuration
## Write the search path of the openssl library file
echo “/usr/local/openssl/lib” >> /etc/ld.so.conf
## To make the modified /etc/ld.so.conf take effect
ldconfig -v
# 6. Check the openssl version
openssl version
openssl version note:
/usr/local/openssl/bin/openssl: error while loading shared libraries: libssl.so.1.1: cannot open shared object file: No such file or directory
If your libssl.so.1.1 file is under /usr/local/openssl/lib/, you can do this
ln -s /usr/local/openssl/lib/libssl.so.1.1 /usr/lib64/libssl.so.1.1
ln -s /usr/local/openssl/lib/libcrypto.so.1.1 /usr/lib64/libcrypto.so.1.1
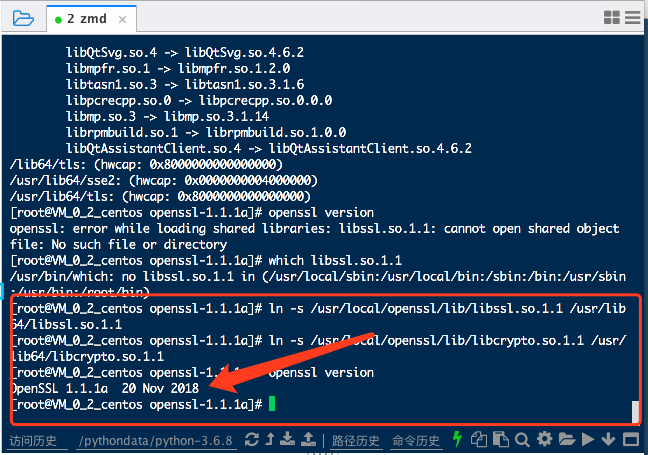
3.3 reinstall python
./configure –prefix=/usr/local/python3 –with-openssl=/usr/local/openssl
make
make install