About TypeError:strptime()argument1mustbestr,notbytes Explanation
When using datetime.strptime(s,fmt) to output the result date result, an error occurs
TypeError:strptime()argument1mustbestr,notbytes
My source code is as follows
def datestr2num(s):
return datetime.strptime(s,“%d-%m-%Y”).date().weekday()
dates=np.loadtxt(‘data.csv’,delimiter=’,‘,usecols=(1,),converters={1: datestr2num},unpack=True)
data.csv:
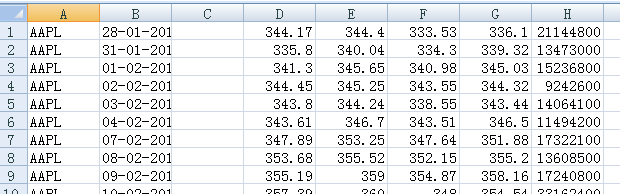
When the compiler opens the data.csv file, the second column of array values in the table is extracted and returned to dates, the second column of values is a date format string, but because we are opening the second column of values in binary encoding format is, the returned value bytes string bytes, so it needs to be string, then the string is decoded with the function decode(‘asii ‘), into string format.
def datestr2num(s):
return datetime.strptime(s.decode(‘ascii’),“%d-%m-%Y”).date().weekday()
dates=np.loadtxt(‘data.csv’,delimiter=’,‘,usecols=(1,),converters={1: datestr2num},unpack=True)
line
is a bytestring,because you opened the file in binary mode. You’ll need to decode the string; if it is a date string matching the pattern,you can simply use ASCII:
time.strptime(line.decode('ascii'), '%Y-%m-%d ...')
You can add a'ignore'
argumentto ignore anything non-ASCII,but chances are the line won’t fit your date format then anyway.
Note that you cannot pass a value that containsmorethan the parsed format in it; a line with other text on itnotexplicitly covered by thestrptime()
pattern willnotwork,whatever codec you used.
And if your input really varies that widely in codecs,you’ll need to catch exceptions one way or another anyway.
Aside from UTF-16 or UTF-32,I wouldnotexpect you to encounter any codecs that use differentbytesfor the arabic numerals. If your input really mixes multi-byte and single-byte codecs in one file,you have a bigger problem on your hand,notin the least because newline handling willbemajorly messed up.