The purchased ECS runs the yum command, and the yum command not found appears.
By uninstalling Yum and python from the virtual machine, you need to pay attention to the first line of the/usr/bin/Yum file. I define it as “#!/usr/bin/Python”
1. Uninstall Python:
1 rpm -qa|grep python|xargs rpm -e --allmatches --nodeps
2 whereis python|xargs rm -fr
2. Uninstall Yum:
1 rpm -qa|grep yum|xargs rpm -e --allmatches --nodeps
2 rm -rf /etc/yum.repos.d/*
3 whereis yum|xargs rm -fr
3. Create directory Python and Yum to store RPM package:
1 mkdir /usr/local/src/python
2 mkdir /usr/local/src/yum
4. Use WGet to download the RPM package of Python and Yum respectively (Note: it must correspond to the version number of the system)
(1) Download the RPM package of Python:
1 #cd /usr/local/src/python
2
3 #wget http://vault.centos.org/7.2.1511/os/x86_64/Packages/python-2.7.5-34.el7.x86_64.rpm
4 #wget http://vault.centos.org/7.2.1511/os/x86_64/Packages/python-iniparse-0.4-9.el7.noarch.rpm
5 #wget http://vault.centos.org/7.2.1511/os/x86_64/Packages/python-pycurl-7.19.0-17.el7.x86_64.rpm
6 #wget http://vault.centos.org/7.2.1511/os/x86_64/Packages/python-devel-2.7.5-34.el7.x86_64.rpm
7 #wget http://vault.centos.org/7.2.1511/os/x86_64/Packages/python-libs-2.7.5-34.el7.x86_64.rpm
8 #wget http://vault.centos.org/7.2.1511/os/x86_64/Packages/python-urlgrabber-3.10-7.el7.noarch.rpm
9 #wget http://vault.centos.org/7.2.1511/os/x86_64/Packages/rpm-python-4.11.3-17.el7.x86_64.rpm
(2) Download RPM package from Yum:
1 #cd /usr/local/src/yum
2
3 #wget http://vault.centos.org/7.2.1511/os/x86_64/Packages/yum-3.4.3-132.el7.centos.0.1.noarch.rpm
4 #wget http://vault.centos.org/7.2.1511/os/x86_64/Packages/yum-metadata-parser-1.1.4-10.el7.x86_64.rpm
5 #wget http://vault.centos.org/7.2.1511/os/x86_64/Packages/yum-plugin-fastestmirror-1.1.31-34.el7.noarch.rpm
5. Install the RPM package of Python and yum
(1) Install Python:
1 #cd /usr/local/src/python
2
3 #rpm -ivh python-* rpm-python-*
4 Dependency problems with the installation package occur, at which point the following solution can be found.
5
6 #rpm -ivh python-* rpm-python-* --nodeps --force
7 --nodeps --force is to force installation regardless of dependencies.
8
9 Once installed, you can run python:
(2) Install Yum:
1 #cd /usr/local/src/yum
2 #rpm -ivh yum-*
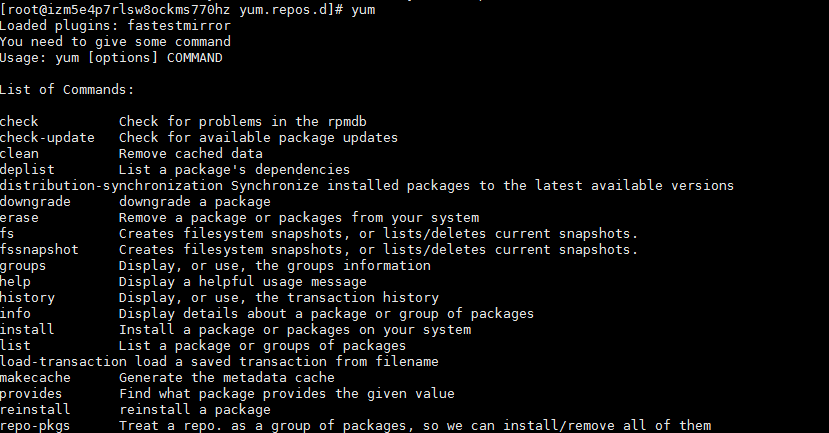