Error when starting a django project
C:\Users\jerry.home-pc\PycharmProjects\mysite>python manage.py startapp app01
Traceback (most recent call last):
File “manage.py”, line 22, in <module>
execute_from_command_line(sys.argv)
File “C:\Python27\lib\site-packages\django\core\management\__init__.py”, line 363, in execute_from_command_line
utility.execute()
File “C:\Python27\lib\site-packages\django\core\management\__init__.py”, line 337, in execute
django.setup()
File “C:\Python27\lib\site-packages\django\__init__.py”, line 27, in setup
apps.populate(settings.INSTALLED_APPS)
File “C:\Python27\lib\site-packages\django\apps\registry.py”, line 108, in populate
app_config.import_models()
File “C:\Python27\lib\site-packages\django\apps\config.py”, line 202, in import_models
RuntimeError: maximum recursion depth exceeded while calling a Python object
Command line can not be started, pycharm also can not be started
Analyze the cause: check the system python version number:.
C:\Users\jerry.home-pc\PycharmProjects\mysite>python -V
Python 2.7
Solution: Upgrade python to 2.7.5 (referenced from: https://stackoverflow.com/questions/16369637/maximum-recursion-depth-exceeded-in-cmp-error-while-executing-python-manage-py-r?rq=1)
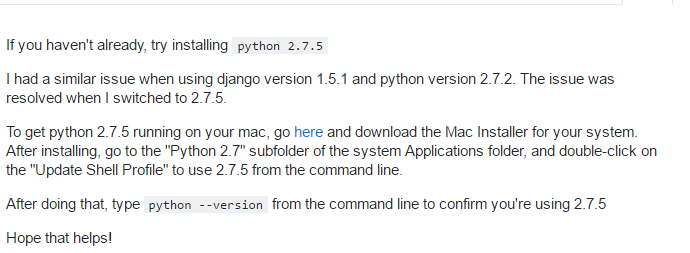
To view the Python version number after upgrading.
C:\Users\jerry.home-pc\PycharmProjects\mysite>python -V
Python 2.7.5
Start successfully after upgrade:
C:\Users\jerry.home-pc\PycharmProjects\mysite>python manage.py startapp app01
Method 2: modify the functools.py file to achieve the same effect (not tested, you can try it yourself if you are interested)