Tool cracking
Two days ago, a wave of project cases came down on the Internet, and all of them turned out to be encrypted compressed packages, so I went to the Internet to find a tool to crack compressed packages.
it took a long time for one compressed package to crack. After decompressing three compressed packages, I gave up and prepared to find another method
Password dictionary
Coincidentally, the three cracked codes are all 4-digit codes, which reminds me to rely on the dictionary to crack
do as you say, and reach out to
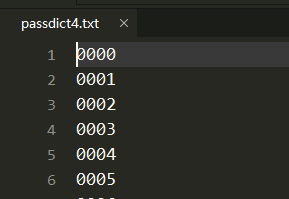
f = open('passdict4.txt','w')
for id in range(10000):
password = str(id).zfill(4)+'\n'
f.write(password)
f.close()
Sure enough, the guess is right, and the cracking speed is really fast.
Xi dada said, Science and technology is the first productivity, and innovation is the first driving force leading development.
since there are faster methods, why not study an automatic method
Zipfile Library
Python has a library called zipfile, which can extract zip files from its related documents
ZipFile.extractall (path = none, members = none, PWD = none)
unzip the specified file in the zip document to the current directory.
The parameter path specifies the folder where the parsing file is saved
the parameter members specifies the name of the file to be decompressed or the corresponding zipinfo object
the parameter pwd is the decompression password.
Just loop through the zip files in the folder and unzip them one by one.
note: there is a Chinese file name in Python 3 with messy code, which will ZipFile.py “Cp437” in can be changed to “GBK” (two places need to be changed)
z = zipfile.ZipFile(f'{file_path}', 'r'
z.extractall(path=f"{
root})
z.close()
On this basis, add the circular password dictionary
note: since decompression is a password error, an exception will be generated. Here, try except can be used for processing
passFile = open(r"D:\python\passdict4.txt")
for line in passFile.readlines():
password = line.strip('\n')
try:
zip_file.extractall(path=f"{root}", pwd=password.encode("utf-8"))
print(password)
break
except:
pass
zip_file.close()
After such an operation, I thought it would be a great success, but I didn’t expect it to be so simple.
most of the time, it can be cracked, but some of them fail, and the helpless websites find a way to do it.
at first, they thought it was the wrong call of the extractall method, but the error was the wrong password, which made me very fascinated
by this Only when I saw an article in one place did I know
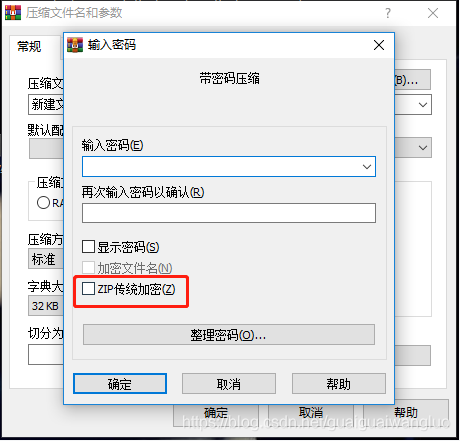
By default, WinRAR uses AES-256 to encrypt zip files in CTR mode, while traditional encryption uses CRC32 encryption, that is, zip 2.0 traditional encryption algorithm, although AES-256 is better than zip 2.0 traditional encryption algorithm is much safer, but it may be compatible with some old decompression software. However, the zipfile module in Python standard library only supports CRC32 encrypted zip files, so it is impossible to decompress through zipfile library without traditional encryption
I’ve spent so much effort, and it’s really too much effort if I give up here.
since the encryption method is different, how can the decompression software be directly decompressed?
here’s an idea. If I can call the decompression software from the code, it’s easy to do.
so I quickly use the stunt
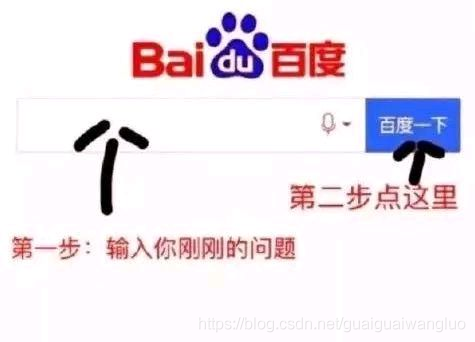
to solve the problem Successful search, 7z and other decompression software has related functions
Calling the third party software command line
Configure environment variables
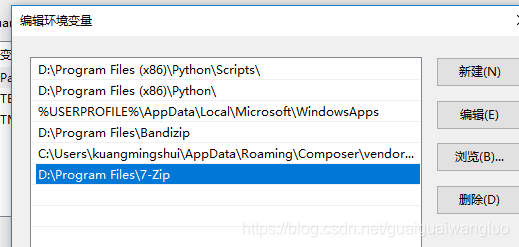
command line verification
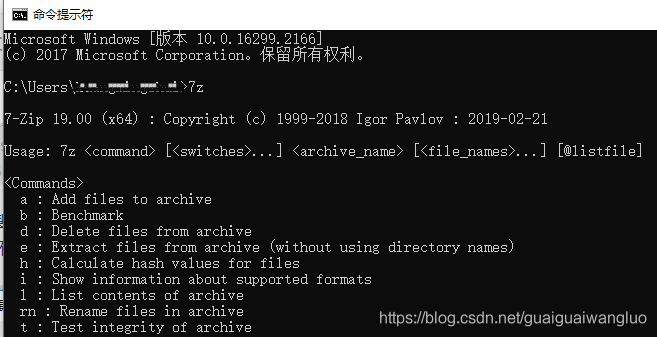
nese, the configuration is successful
passFile = open(r"D:\python\passdict4.txt")
for line in passFile.readlines():
password = line.strip('\n')
# t
command='7z -p'+password+' t '+file_path
child=subprocess.call(command)
if(child==0):
print(password)
break
Different encryption methods are solved, but the greed is really terrible
repeated calls to the command line make me unhappy again
Pyzipper Library (Ultimate)
It happened that when checking the encryption method, I saw someone propose that
Python has a pyzipper library, which can be very compatible instead of zipfile, and can read and write AES encrypted zip files
all come here, there is no retreat
#Install pyzipper
PIP install pyzipper
There will be garbled Chinese name, remember to change it
f1 = open('D:\python\passdict4.txt','r')
with pyzipper.AESZipFile(file_path,'r') as f:
for i in f1:
i = i.rstrip('\n')
f.pwd = str.encode(i)
try:
f.extractall(path=f"{root}")
print(file_path+"\t the password is:"+i)
break
except Exception:
pass
f.close()
f1.close()
This method is perfect
Click to download relevant codes, files and tools