The operation before and after the model is as follows:
First migration:
class Snippet(models.Model):
created = models.DateTimeField(auto_now_add=True)
title = models.CharField(max_length=100, blank=True, default='')
code = models.TextField()
linenos = models.BooleanField(default=True)
language = models.CharField(choices=LANGUAGE_CHOICES, default='python', max_length=100)
style = models.CharField(choices=STYLE_CHOICES, default='friendly', max_length=100)
After change:
class Snippet(models.Model):
created = models.DateTimeField(auto_now_add=True)
title = models.CharField(max_length=100, blank=True, default='')
code = models.TextField()
linenos = models.BooleanField(default=True)
language = models.CharField(choices=LANGUAGE_CHOICES, default='python', max_length=100)
style = models.CharField(choices=STYLE_CHOICES, default='friendly', max_length=100)
owner = models.ForeignKey('auth.User', related_name='snippets', on_delete=models.CASCADE)
highlighted = models.TextField()
There are three steps for Django to modify the database
1. Make changes to models.py (normal)
2. Creating migrations using makemigrations
3. Migration using migrate
Analysis: after the modified model, the code and highlighted fields cannot be empty when the database is synchronized for the second time. If you create the highlighted field the first time, there is no impact because the table does not have an existing row. If the data table has been created for the first time and data has been inserted into the table, a default value must be defined to fill in the existing row, otherwise the database will not accept the data table changes because of violating the integrity of the data
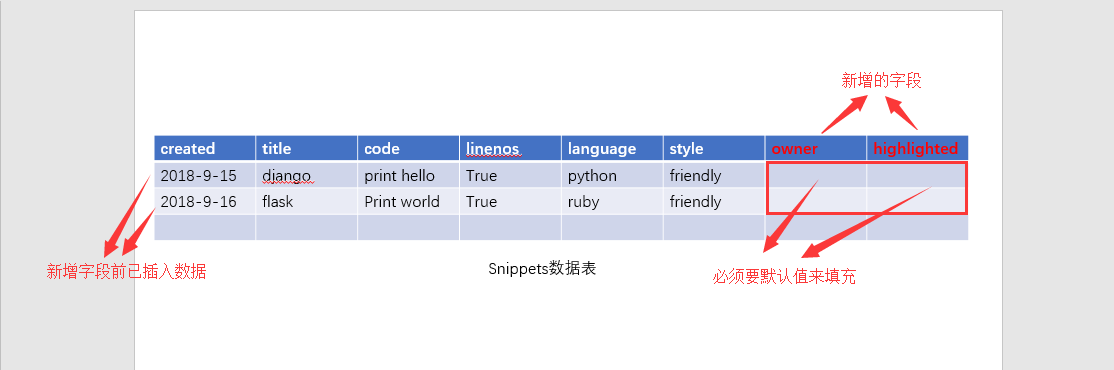
Solution: change to
code = models.TextField(default =”)
highlighted = models.TextField(default =”)