I’m mysql8 For versions above 0, start to report errors when connecting to the MySQL database in the server with nodejs

This means that the server starts up, but there is an error in the password protocol in the database. The result I found on the Internet tells me that it is mysql8.0 supports a new cryptographic protocol, but nodejs does not support it at present.
The following is my solution after avoiding the pit, which may not be applicable to everyone
1. Log in to MySQL server
Open the command line as an administrator and enter
mysql -u administrator -p
If you are prompted that MySQL is not an executable file, you can CD on the command line to the bin directory in mysql, or add Mysql to the environment variable
Note: the above root is the user name you want to modify. It may not be administrator. Mine is administrator
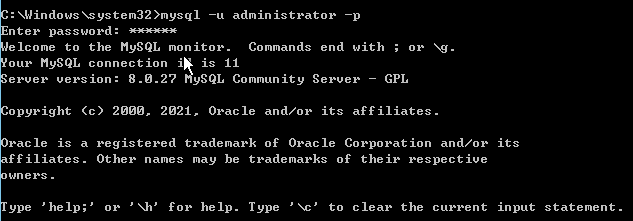
2. Enter the password
Then enter the password and enter mysql
3. Select database
Therefore, first we need to select the database and enter it on the command line
use mysql
Note: This is mysql, not any other name, unless you modify the name of the table in the database where the user name and password are stored, but the probability is very small, and you can’t move there.
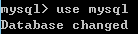
If the wrong database is selected, the following errors will be prompted in the subsequent password blank operation

4. Leave password blank
If the original password is not blank and the password is modified directly, an error will be reported

Password blank operation, enter
update user set authentication_string='' where user='administrator';
The above administrator is still the user name you need to modify the password protocol

5. Refresh
This step is important
flush privileges;

6. Modify password and agreement
I’ve been stuck in this step for a long time
When I modify the password agreement, the error in the figure appears

After checking a lot of information, I understand
Open the database, MySQL database and user table, and you can see
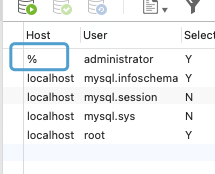
The host before the user name I want to modify is’% ‘instead of’ localhost ‘, so I need to change the localhost in the command to%
alter user 'administrator'@'%' identified with mysql_native_password by '123456';
The above administrator is the user name you need to change your password, and 123456 is your password

This completes all steps
MySQL forgot password modification, etc. many online tutorials don’t seem to apply to MySQL 8 0. Write this part when you have time.