When using Ubuntu, you often encounter such an error prompt: “system program problem detected”
As shown in the figure:
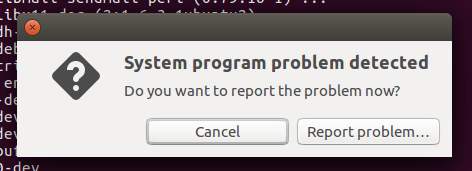
Reason:
There is a built-in utility called app in Ubuntu, which can notify when a program crashes. This program, called import, notifies you of errors whenever you log into your Ubuntu system. It’s normal that you don’t have to panic or be afraid of such a mistake
Solution:
1. Inform system developers to do this. It’s recommended, and it won’t take you long. Click the “report prolem” button in the error prompt dialog box. You will be prompted to enter your administrator account password. Then, click “continue” to send the error prompt to developers, so that they can help solve the problem
First, make sure you are connected to the Internet properly. Once you submit the error report, developers will study the report carefully and try to solve the error in the next release
After submitting the error prompt, you can delete the file with the crash error prompt from your local system. If you want to see these error prompt files, you can enter the following command in the terminal: LS/var/crash/
Output (everyone is different)
libglib2.0-dev.0.crash_ usr_ bin_ appstreamcli.0.uploaded _ usr_ bin_ appstreamcli.0.crash _ usr_ bin_ pycompile.0.crash _ usr_ bin_ Appstreamcli. 0. Upload
delete all through the following command: sudorm – FR/var/crash/*
2. Disable the function of import. This is not recommended
Edit the app file/etc/default/APP
sudo nano /etc/default/apport
Find the line enabled=1 and change it to 0(zero)
# set this to 0 to disable apport, or to 1 to enable it
# you can temporarily override this with
# sudo service apport start force_start=1
enabled=0
Press crtl+x to exit, press y to save (nono), enter
Note: nano is a small, free and friendly editor. There are nano commands on most Linux
Then, you can stop the app service
Sudo stop apport
The app will not report any errors